The TW3Httprequest object is a fairly low level object for talking with servers via the HTTP protocol. As expected you can issue commands like GET and POST in order to retrieve or send data. There are also details like ready-state and status properties to keep an eye on while you work with the object. It is also important to note that all connectivity in the browser is a-sync (non blocking), so you must use the events to handle incoming data.
NOTE: It is extremely important to remember that the browser is bound by something called "the same origin policy". This means that you can’t (unless the server allows it) download media from anywhere you like. The request has to be within the same domain as your application comes from. In short, you can only download things from your own server where the smart app is stored. Some servers allow cross domain downloading (google being a prime example) but there are security issues you must follow when working.
SmartCL.Inet
TW3HttpRequest = class(TObject)
Methods:
public
procedure Get(aURL: String);
procedure Open(aMeth, aURL: String);
procedure SetRequestHeader(headerName, value: String);
procedure Send; overload;
procedure Send(textData: String); overload;
procedure Abort;
function Status: Integer;
function StatusText: String;
function ResponseXML: Variant;
function ResponseText: String;
function ReadyState: THttpRequestReadyState;
Properties:
property Handle: THandle read FReqObj;
property RequestHeaders[headerName: String]: String write SetRequestHeader;
property OnDataReady: THttpRequestEvent read FOnDataReady write FOnDataReady;
property OnReadyStateChange: THttpRequestEvent read FOnReadyStateChange write FOnReadyStateChange;
property OnLoad: THttpRequestEvent read FOnLoad write FOnLoad;
property OnError: THttpRequestEvent read FOnError write FOnError;
Downloading a HTML document
Downloading a HTML document from a website (which of-course can be a PHP function or a REST method) is very straightforward. In this example I am using a visual application that has a form. So create a new visual project, add a button and a memo to the form – then type in the event handlers below:
type
TForm1 = class(TW3Form)
private
{ Private methods }
{$I 'Form1:intf'}
FHttp: TW3HttpRequest;
procedure HandleButtonClick(Sender:TObject);
procedure HandleHttpDataReady(Sender:TW3HttpRequest);
procedure HandleHttpReadyStateChanged(Sender:TW3HttpRequest);
protected
{ Protected methods }
procedure InitializeObject; override;
procedure FinalizeObject; override;
procedure StyleTagObject; reintroduce; virtual;
procedure Resize; override;
end;
Setting up the HTTP request
In the constructor we initialize the HTTP object so that everything is ready for use. In this example I have a couple of controls on the form, so just ignore that part if you like.
procedure TForm1.InitializeObject;
begin
inherited;
{$I 'Form1:impl'}
AdjustToParentBox;
W3HeaderControl1.BackButton.Visible := False;
W3HeaderControl1.Title.Caption := 'Network test';
(* Setup the http request object *)
FHttp := TW3HttpRequest.Create;
FHttp.OnDataReady := HandleHttpDataReady;
FHttp.OnReadyStateChange := HandleHttpReadyStateChanged;
(* Add our event handler to Button1 *)
W3Button1.OnClick := HandleButtonClick;
end;
Taking care of events
procedure TForm1.HandleButtonClick(Sender:TObject);
begin
(* Try to download the html from google.com *)
FHttp.Get('http://www.google.com');
end;
procedure TForm1.HandleHttpReadyStateChanged(Sender:TW3HttpRequest);
begin
(* Just for watching the status codes *)
if Sender.ReadyState=4 then
begin
WriteLn(Sender.Status);
WriteLn(Sender.ReadyState);
end;
end;
procedure TForm1.HandleHttpDataReady(Sender:TW3HttpRequest);
begin
(* Document is downloaded, now display the text in our memo *)
w3memo1.Text := FHttp.ResponseText;
end;
The end result is as expected:
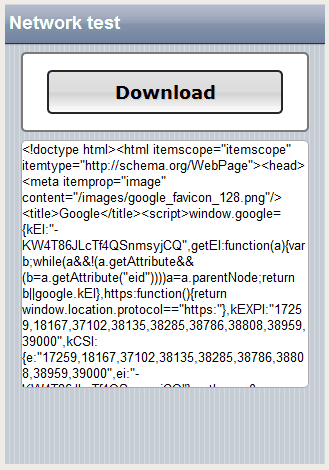
Downloading HTML is easy
|